Dropdown field tracking in Google Analytics 4 using Google Tag Manager
In some cases, you might need to know if certain dropdown fields are being used (e.g. product filters) and maybe even see what values are selected most often. Today we will set up dropdown field tracking in Google Analytics 4 with a help of Google Tag Manager.
Since GTM doesn’t track dropdowns (select fields) by default at the moment, we will use a small JavaScript snippet that I prepared earlier. This snippet will listen for any changes in all (or specific) dropdowns on the website and pass relevant information to the Data Layer.
Custom HTML code snippet
To make it easier we will use a simple code snippet below to capture any changes to dropdown fields and do 2 things:
- Send data layer event when a new value is picked from the dropdown – “dropdownChanged”
- Send information about the field name and selected value.
These values will be fetched by Google Tag Manager and sent to Google Analytics 4, and you can use it to pass information to any other tool.
<script>
(function(){
var dropdowns = document.querySelectorAll('select');
for(var i=0; i <= dropdowns.length - 1; i++) {
dropdowns[i].addEventListener('change', function(e) {
dataLayer.push({
'event': 'dropdownChanged',
'fieldValue': e.target.options[e.target.selectedIndex].text,
'fieldName': e.target.name
});
});
}
})();
</script>
How does it work?
First, we are selecting all elements from the page with <select> tag:
var dropdowns = document.querySelectorAll('select');
Next, we are listening for a “change” event for all matched dropdowns (select fields):
dropdowns[i].addEventListener('change', function(e) {
After that, we send additional information to dataLayer if a dropdown value is changed. In addition to ‘dropdownChanged’ event we are passing the dropdown name and selected value text:
dataLayer.push({
'event': 'dropdownChanged',
'fieldValue': e.target.options[e.target.selectedIndex].text,
'fieldName': e.target.name
});
Limitations
Note that this template will work out of the box only for default dropdown fields that look similar to this:
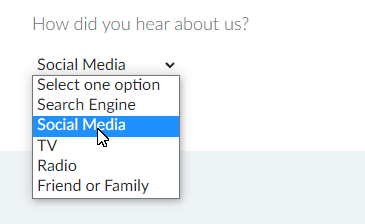
And this is how those fields usually look in HTML code:
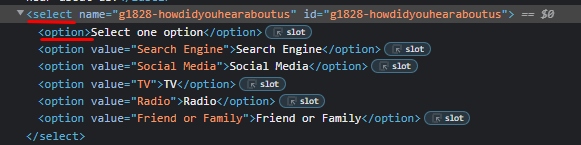
For dropdown fields that are altered/created using 3rd party plugins this code might not work, since there might be no <select> field at all in the background or it is not updated the same way as a regular dropdown. For those cases, you might need to set up click events or use a custom data layer event to capture required information.
Create a custom HTML tag
Copy the code above and create a new Tag with the Type “Custom HTML”. If you want to collect dropdown events from all website pages you can use the “All Pages” trigger, but normally you would want to limit this tag only to specific pages where necessary dropdown fields can be found.
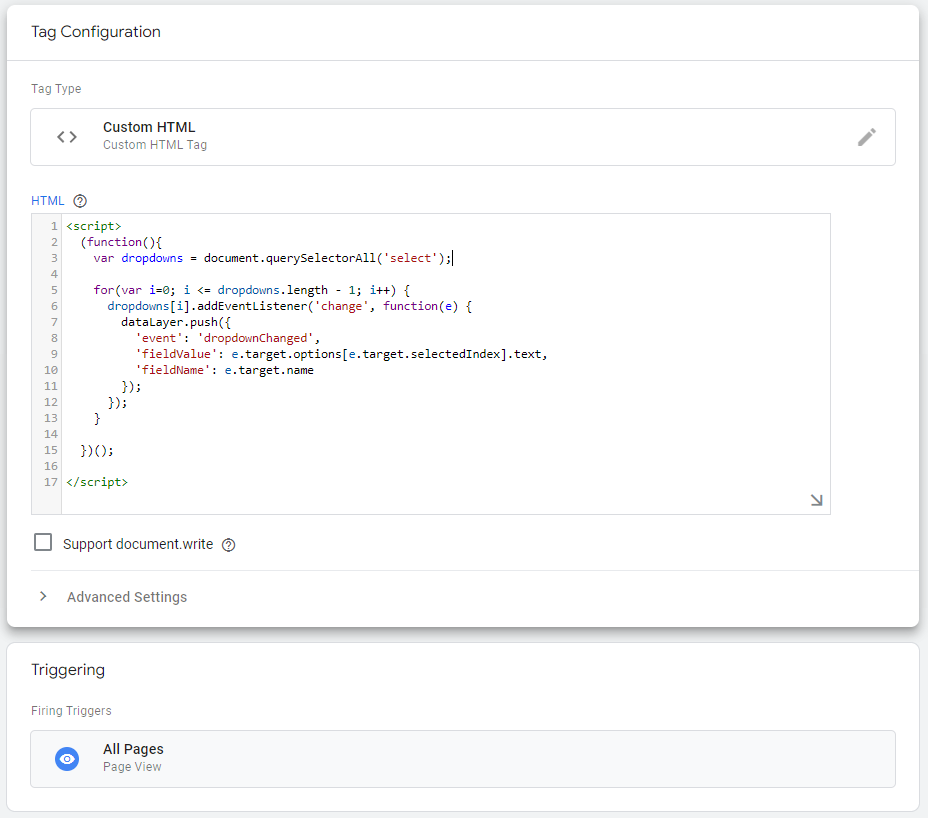
Extract the dropdown name and value from the data layer
To get the dropdown name and value from the data layer we will need to create additional variables in GTM. Go to the Variables section and create 2 new Data Layer variables:
- fieldValue
- fieldName
It is important to use exactly the same name as in the JS code snippet since these values are case-sensitive.
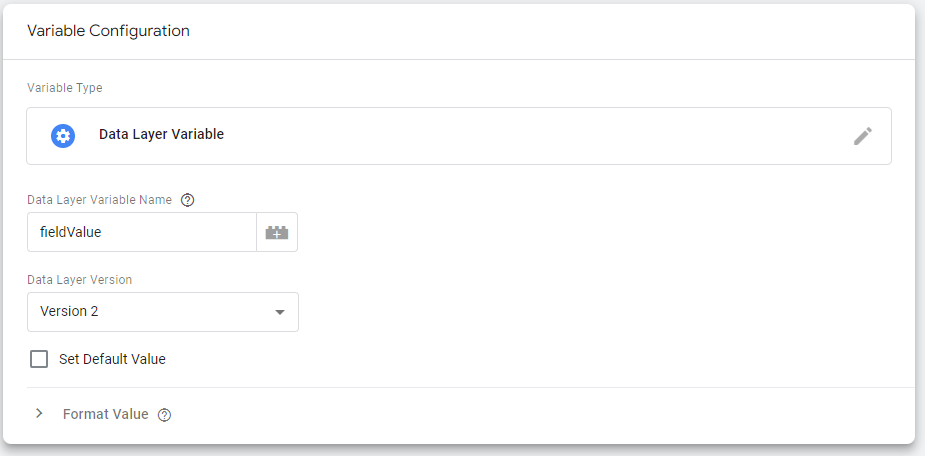
Create Google Analytics 4 event tag
To enable dropdown field tracking in Google Analytics 4 add a GA4 event tag that will collect dropdown change events and field values when data layer receives this data.
Create a new tag with the type “GA4 Event”, select your GA4 configuration tag, and enter the event name that you want to see in your reports. I will be using “dropdown_change” in this example.
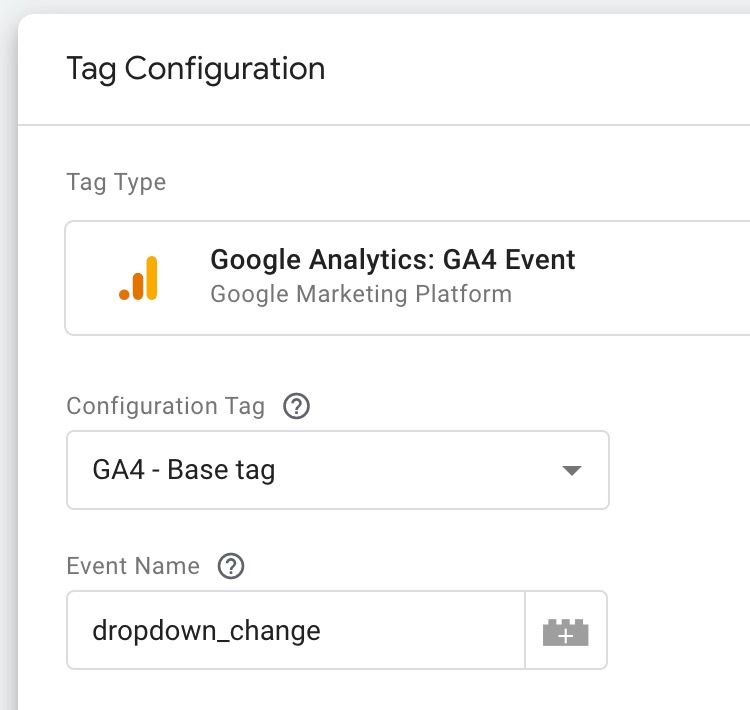
We want to pass the field name and selected dropdown value to Google Analytics 4 so let’s add two additional event parameters:
- field_name
- field_value
As event parameter values we will use corresponding data layer variables that we have just created:
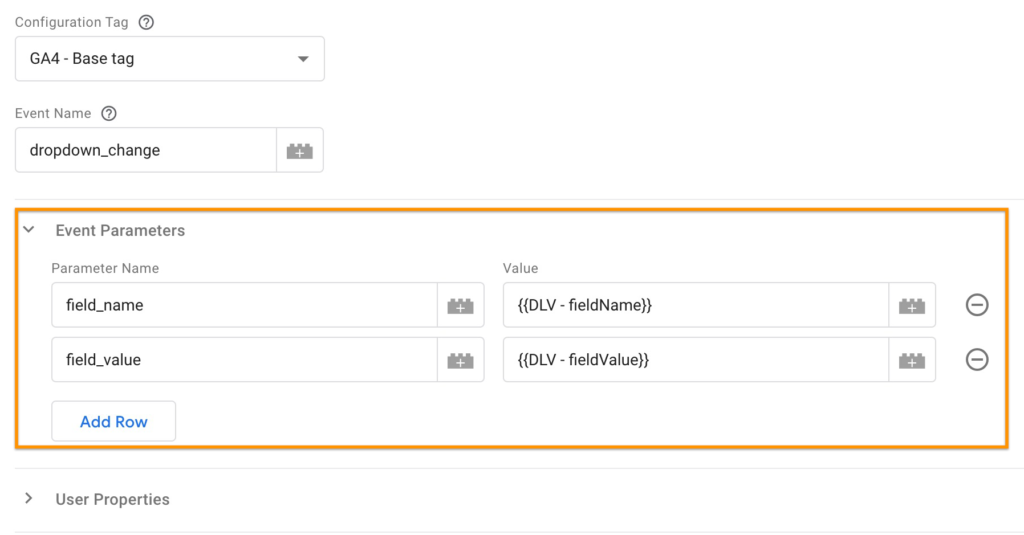
Note that you will need to create custom dimensions “field_name” and “field_value” in Google Analytics 4 interface, otherwise this data won’t be collected. Alternatively, you can use any of your existing custom dimensions as event parameters in the GA4 event tag.
When creating custom dimensions in GA4 (In Admin > Custom definitions section) make sure to use Event scope and exactly the same Event parameter name as in the GTM tag.
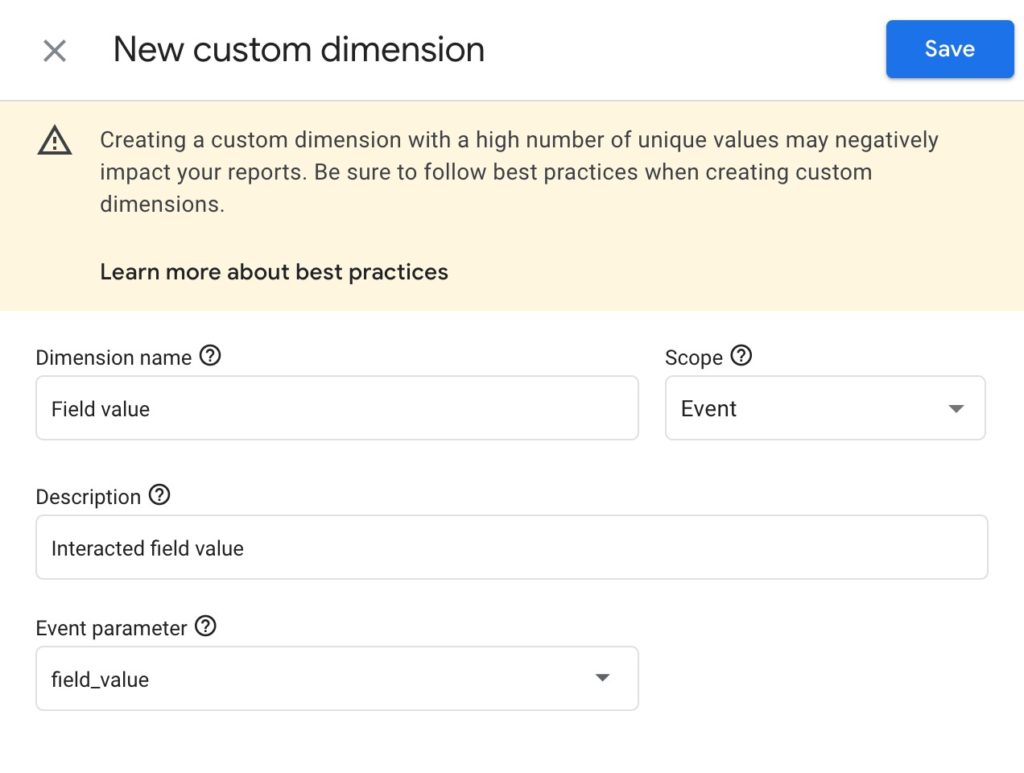
Las thing we need to add here is a trigger, so click on “Triggering” and then create a new trigger.
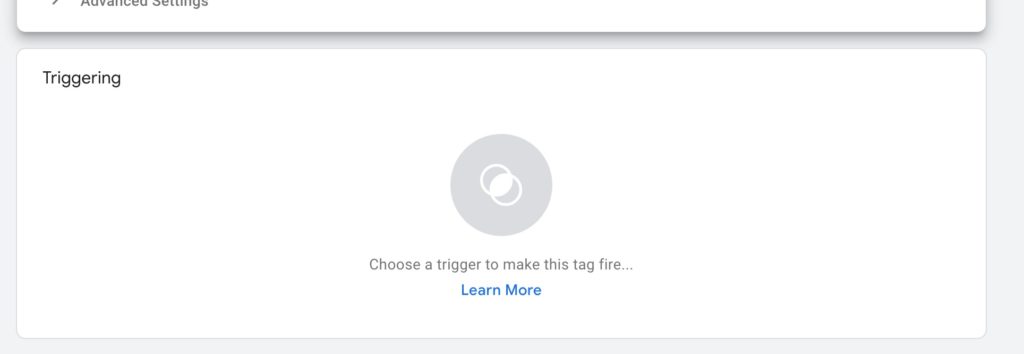
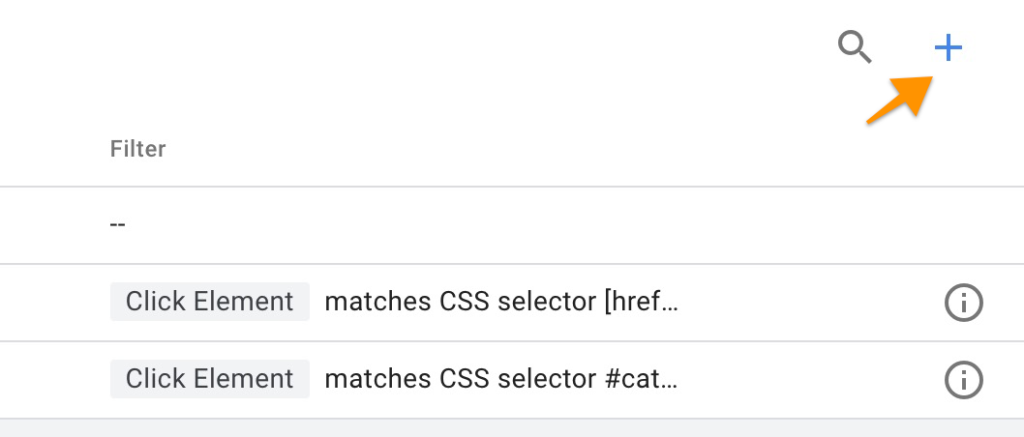
Now select Custom event type and use “dropdownChanged” as the event name, since we have it in our data layer code that we added in a custom HTML tag.
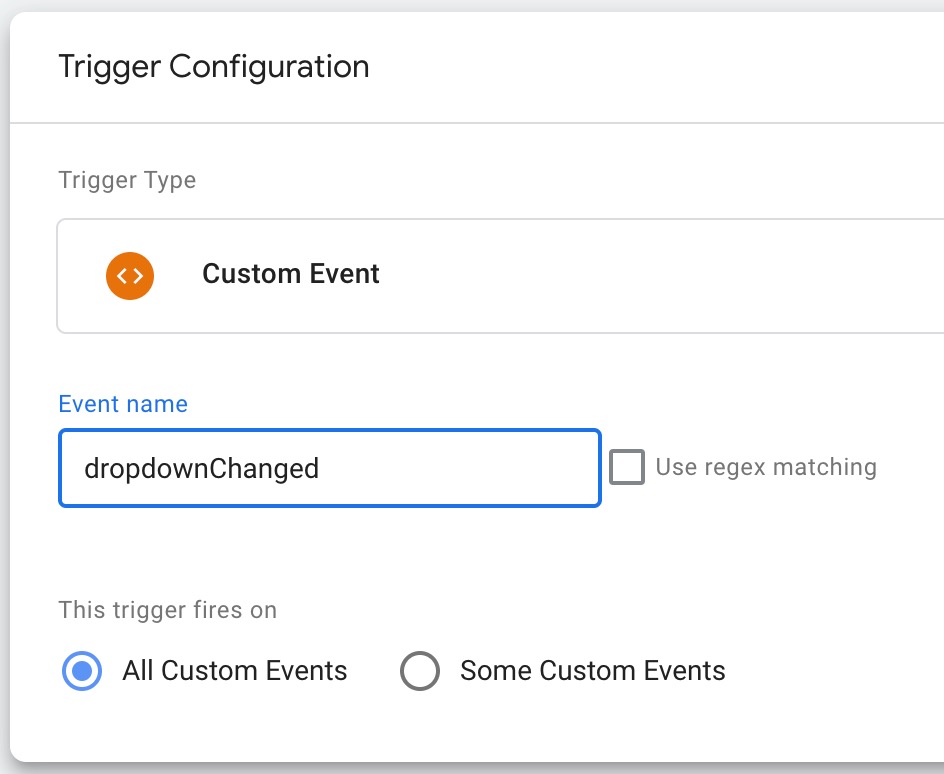
Once you save this it should be attached to your Google Analytics 4 event tag. Save your tag and we are ready to test it.
Testing Dropdown field tracking in Google Analytics 4 and GTM
Enable Preview mode in Google Tag Manager and open a page that contains dropdown (select) fields. You should see a popup in the bottom right corner if the preview has been enabled successfully.
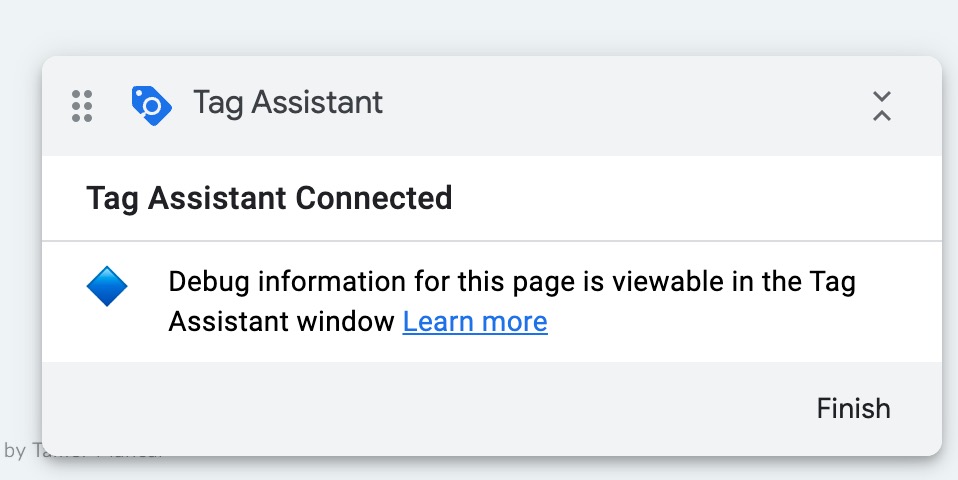
Change values in your dropdown a couple of times and then open the Tag Assistant tab/window to check incoming data layer events.
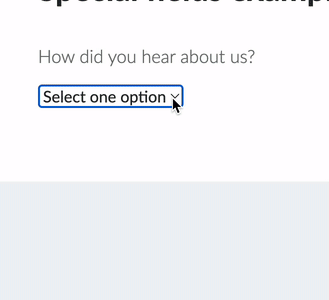

In the events panel on the left side, you should now see “dropdownChange” for each interaction. If you click on the event name you will see exact data layer values as well. In my example, I have “Radio” as my field value and “g1828-howdidyouhearaboutus” as my field name.
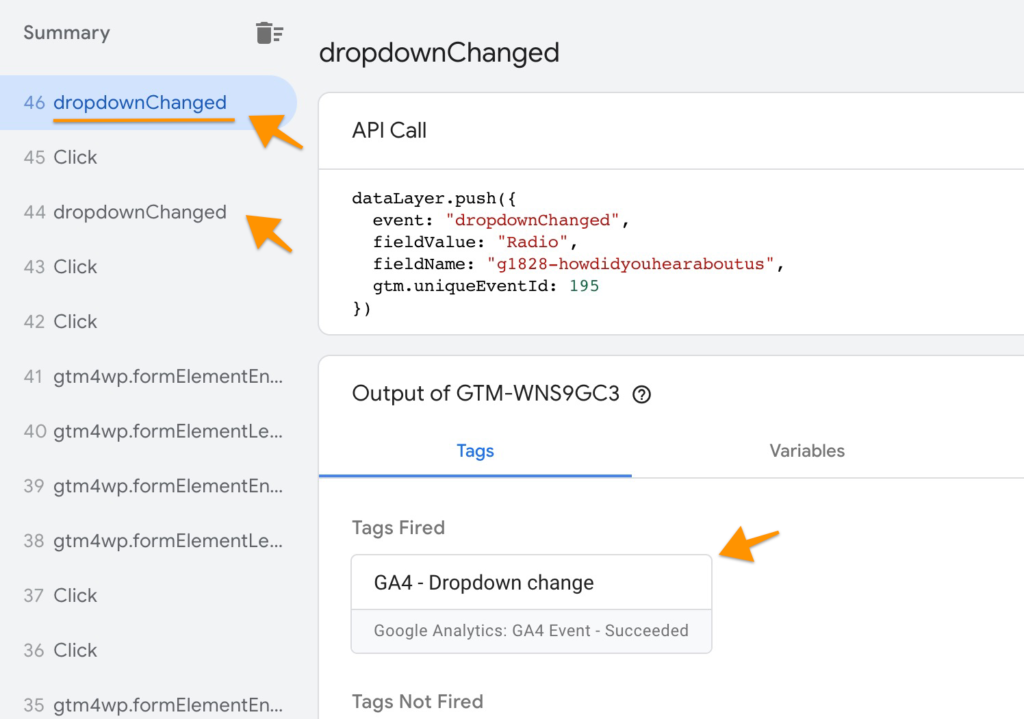
If everything worked as expected you should also see your Google Analytics 4 tag firing when a dropdown value has changed.
Note that dynamic values are passed to the data layer using your HTML structure:
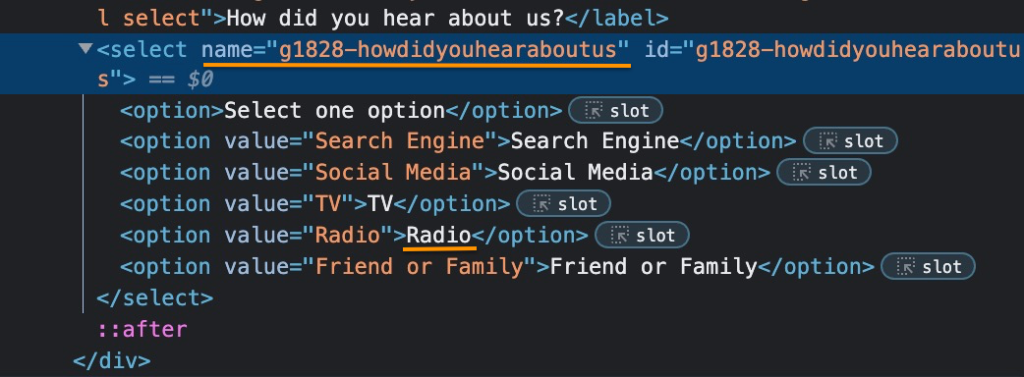
If you would like to use other attributes for your dropdown name and selected value you will need to adjust the custom HTML script accordingly.
To make sure event parameters are reaching Google Analytics as expected you can preview them in GA4 DebugView as well. You should see the same values as in Tag Assistant.
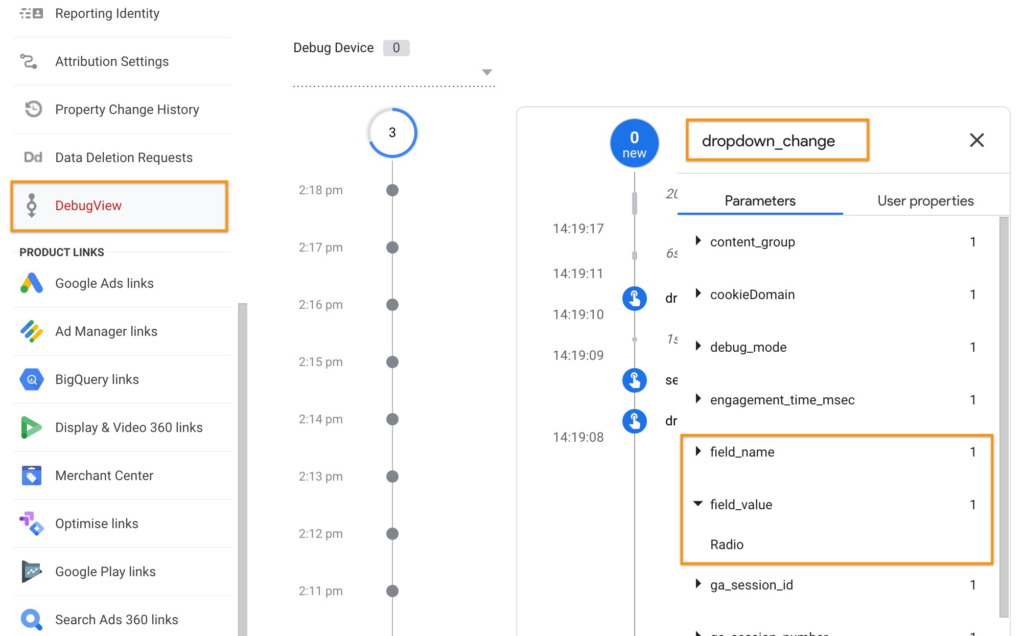
If everything works as expected just publish your Google Tag Manager container and you are good to go!
Troubleshooting common issues
- I can’t see any data layer events when the dropdown value is changed
Double-check if all of the following are true:
1) Custom HTML tag is firing on the page that has dropdown (You can check that in GTM preview mode).
2) You are tracking the standard dropdown fields as in the examples above. Non-standard fields that only imitate <select> field behavior might not trigger a “change” event when a value was picked. To solve that you might need to use click events or a different custom javascript template that is suited for your structure
3) The form and the dropdown are not inside an iframe. You will not be able to get any information from a 3rd party iframe unless you have GTM installed there.
If you believe there is another issue with your scenario let me know in the comments!
- I have a data layer event but the field name and/or selected dropdown value is not correct.
JS template is using <select> field name and clicked option text as values. Usually, those are present in default dropdowns, but you can inspect your dropdown to see if that’s the case.
You can change what values you are using for the name/value by modifying this part of the code:
'fieldValue': e.target.options[e.target.selectedIndex].text,
'fieldName': e.target.name
For example, to get value from input “value” field instead of text and field name from ID instead of “name” attribute, you could change this to the following:
'fieldValue': e.target.value,
'fieldName': e.target.getAttribute("id")
- I have the correct values in the data layer but by GA4 tag won’t fire
Double-check if the custom event trigger that you have added for the GA4 event tag is exactly the same as was used in dataLayer – “dropdownChanged“
View dropdown performance in Google Analytics 4 reports
To see how your dropdowns were used and what values were picked you can create a simple report using 2 custom dimensions that we created earlier.
Create a new custom exploration in Google Analytics 4 and import at least 3 dimensions – Event name, Field name, and Field value (same custom dimensions that you have created).
Additionally, add Total Users and any other metrics that you would like to see for selected dropdown values.
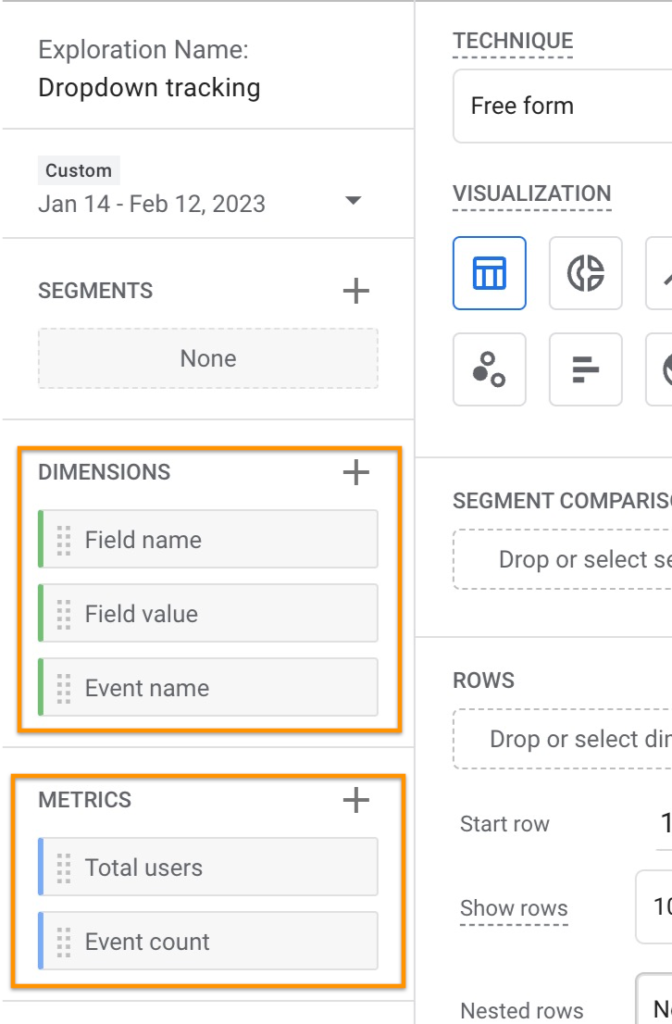
Then, under Tab settings scroll down to the Filters section and filter your data by Event name = “dropdown_change” (or event name that you have used).
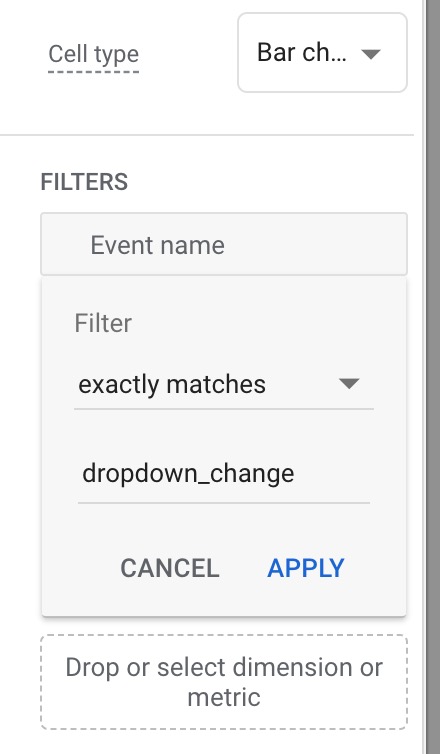
Now, double-click on dimension and metric names to add them to the report (Field name, Field value, Total Users) and the report should be loaded on the right side of your exploration.
Note that you might see your data in the exploration report only 1-2 days after you first started tracking so it won’t appear here right away.
Summary
As you can see is relatively simple to track any standard dropdown interactions in Google Analytics 4 using provided JS template. It might get a bit trickier when your forms are using non-standard dropdowns as implementation will vary depending on its structure. We might cover the most common approaches for other dropdown types in separate blog posts in the future.
Good luck and let me know in the comments if you have any questions!
just give a class to the option and track that class onclick, no extra hassle necessary
In some cases that might work, however this wouldn’t be efficient in all scenarios. Not all of the dropdowns will work if you use just CSS selector with Click trigger and extracting dynamically selected values might be more time consuming.
But of course, if that works and makes most sense in your scenario – the easiest approach is the way to go.
Hi, Thank you so much for this helpful tutorial. Hope you’ll upload a video version.
Hi Mate,
Thanks for this wonderful content on how to track drop-down fields in ga4 via gtm. I’ve implemented the js code and it was tracking good enough. But, the problem araised when one of my form had two drop down fields and when the form was submitted only one drop down field value was tracking the other field value wasn’t showing in ga4. Can you let me know what I need to do here? Thanks!